フランスの生成 AI 企業のトップである Mistral 社が、画像認識にも対応したマルチモーダル モデルの Pixtral 12B を Apache 2.0 ライセンスでリリースしています。画像を読み込ませると、何が映っているかを答えてくれるタイプの AI ですね。こちらを、ボク得意の Mac ローカルオンリー環境で実行した方法を共有します。今回使ったのは、LM Studio と Dify です。Pixtral を実行するだけなら LM Studio の 0.3.5 以上で OK ですが、Dify から API 経由で LM Studio を利用してみたので、後半にはその設定方法を紹介してます。
環境について
Mac
ボクの M2 Max Mac Studio には 32GB しか RAM が積まれていないので、Dify だけは別の M1 Mac Mini で動かしています。これから Mac (M4 搭載 Mac Mini とか MacBook Pro とかいいですな〜) を買う方でローカル LLM も試したいと考えている方は、頑張って 64GB 以上の RAM (ユニファイドメモリ) を搭載した機種を買いましょう。それより少ない RAM 容量で LLM を楽しむのはいろんな工夫や妥協が必要で苦労します。本ブログにはそんな切ない記事があふれています。
LM Studio
v0.3.0 で、インターフェイス含め大幅なアップデートがありました (v0.2 シリーズを使っている場合は、新規でインストールが必要)。その後も着々とバージョンアップが進められ、API 経由で他のアプリから LLM を利用できるようになり、Mac の GPU 用に最適化された MLX バージョンの LLM が動くようになり、v.0.3.5 で Pixtral に対応しました。また、同バージョンでは、メモリ喰い気味の GUI を閉じてサーバだけ動かす Headless mode にも対応したということで興奮したのですが、実際は LLM (pixtral-12B@4bit) がロードされると LM Studio Helper が 12~20GB ほどメモリを使用し続けるので、現状はまだ Ollama のような使い勝手は望めません。残念。
その他 LM Studio v0.3.5 の詳細はこちらをどうぞ: https://lmstudio.ai/blog/lmstudio-v0.3.5
Ollama
2024年 11月 4日現在、Ollama では Pixtral がサポートされていません。Llama 3.2 vision が動くらしい v0.4.0 は pre-release されているので、正式リリースや Pixtral のサポートも時間の問題かもしれません。
Ollama v0.4.0 に関してはこちらをどうぞ: https://github.com/ollama/ollama/releases/tag/v0.4.0-rc6
Dify
これから始める方は最新版になっちゃうんでしょうが、最近、リリース直後は多くのバグで悩むケースが散見されています。ボクはまだ v0.9.1-fix1 を使用しているため、v0.10 以降のファイル添付方法が変わったバージョンでは見た目や使い方が違う可能性があります。Dicord での情報収集が良いと思います。
LM Studio で MLX 版 Pixtral を動かす
LM Studio v0.3.5 以上のインストールは完了してる前提で
無料の Mac 用アプリがここからダウンロードできます。
Pixtral 12B 4-bit をインストール
32GB RAM の Mac では 4-bit (量子化) 版をオススメします。LM Studio 込みでオンメモリで動きます。もっと RAM を積んでいる方は、お好みで 8bit や bf16 版をどうぞ。
虫眼鏡アイコンの Discover → 検索窓に「pixtral」と入力 → MLX だけにチェックを入れる (GGUF を外す) → モデル名やファイルサイズを確認 → Download ボタンクリック
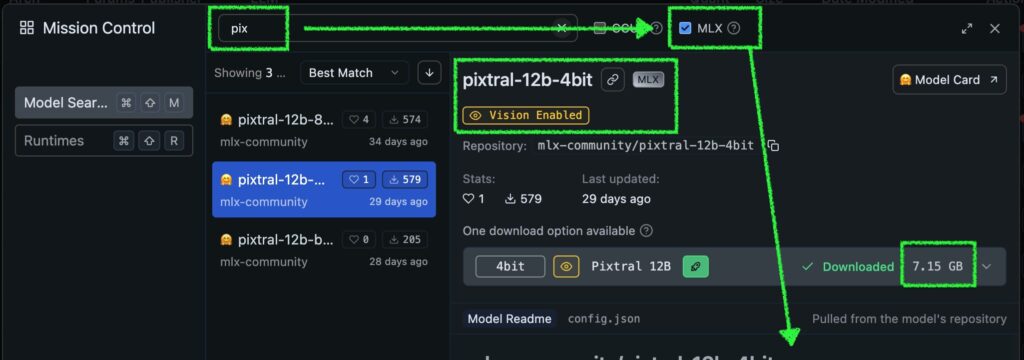
ボクのインターネット環境の問題かわかりませんが、ダウンロード中何度もタイムアウトしていました。左下のボタンでたまに確認し、下のような Timed-out が出ていたら再読み込みボタンをクリックしましょう。
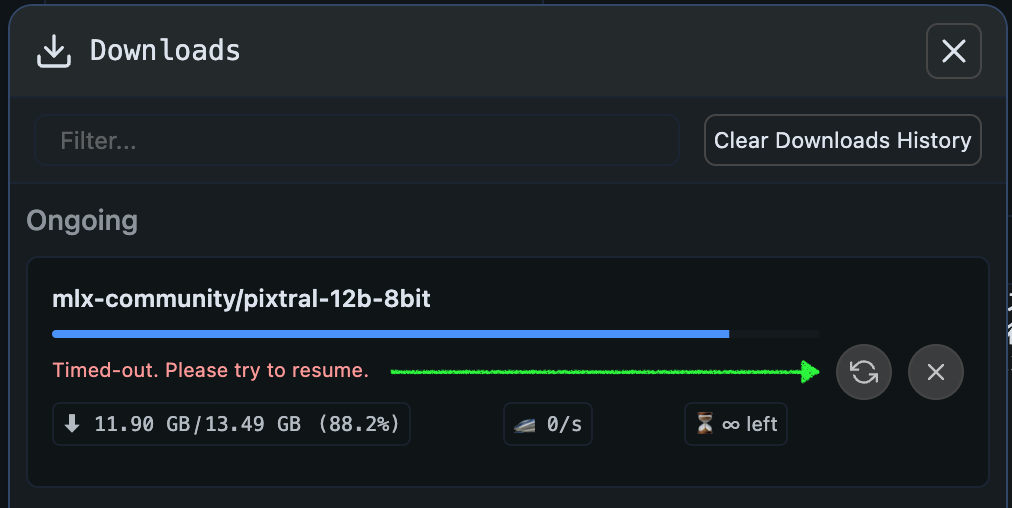
他のエラーで止まることもありますが、再読込で進められます
Chat で試す
Dify で使う必要が無い方 (え?みんなそう?) は、そのまま LM Studio で Pixtral が楽しめます。
吹き出しアイコンの Chat → Select a model to load ドロップダウンから、ダウンロードした Pixtral を選択 → Load Model ボタンクリック
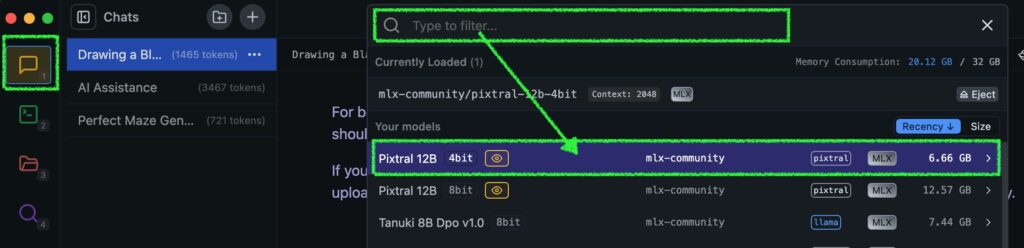
例として、以前没にしたトップ絵をドラッグアンドドロップして「この画像について教えてください」としたところが以下画像です。Pixtral は Mistral Nemo 12B をベースにしていると言うことで、上手な日本語でやりとりができます。ちなみに、Nejumi さんのリーダーボードでは、Mistral Nemo 12B は elyza/Llama-3-ELYZA-JP-8B より日本語性能が上らしいです。確かに。
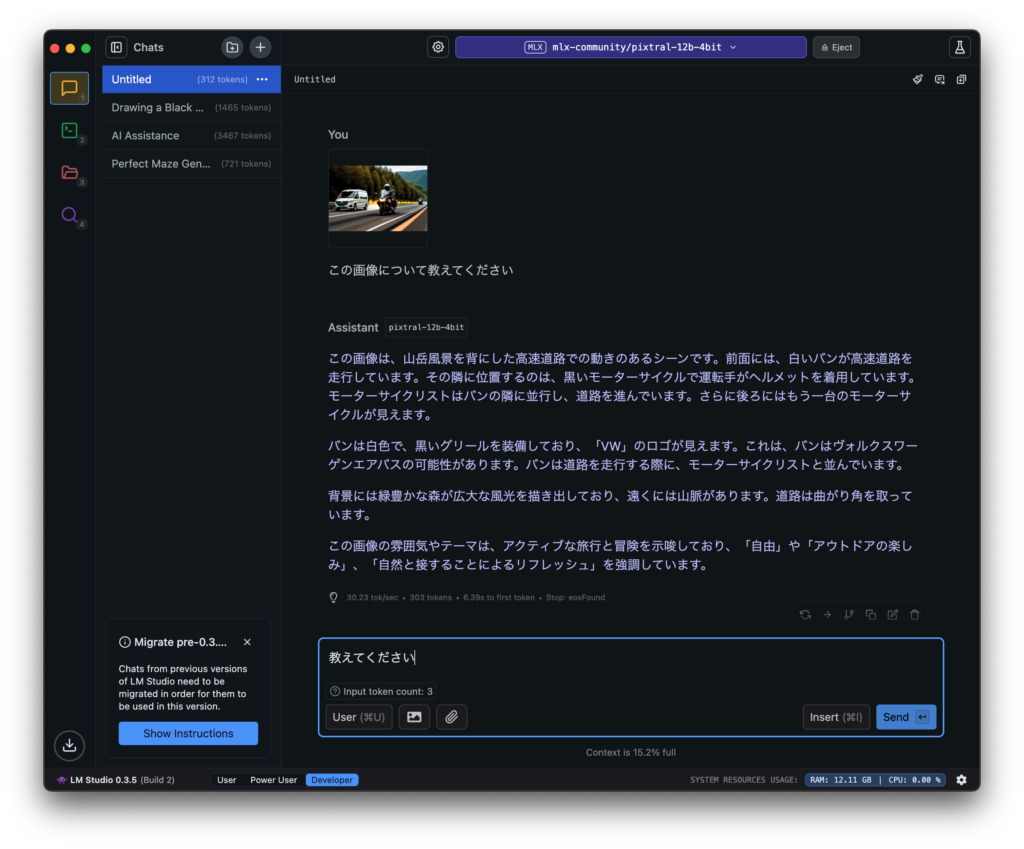
他にもいくつか AI による生成画像を読み込ませてみたところ、大体 30 TPS (トークン/秒) で、正しく詳細な回答が得られました。個人的に画像認識 AI の正しい使い道は見つけられていないのですが、想像以上の性能です。
というわけで、Mac のローカル環境で画像認識マルチモーダル LLM を試したいだけ、という方はお疲れ様でした。いろいろとお試しください。
以降は Dify から API で LM Studio のモデルを使う方法になります。
LM Studio API サーバ設定
基本
Developer アイコンから設定します。全ての Off/On スイッチが表示できていないと思うので、まずは Developer Logs の境界線をつかんで下に押し広げましょう。Start ボタンで API サーバが利用できますが、確認しておいた方がよさそうな設定は以下となります。
- Server Port: デフォルトで 1234
- Server on Local Network: 他の Mac から API アクセスするなら、On
- Just-in-Time Model Loading: Dify からモデルを選択したいなら、On
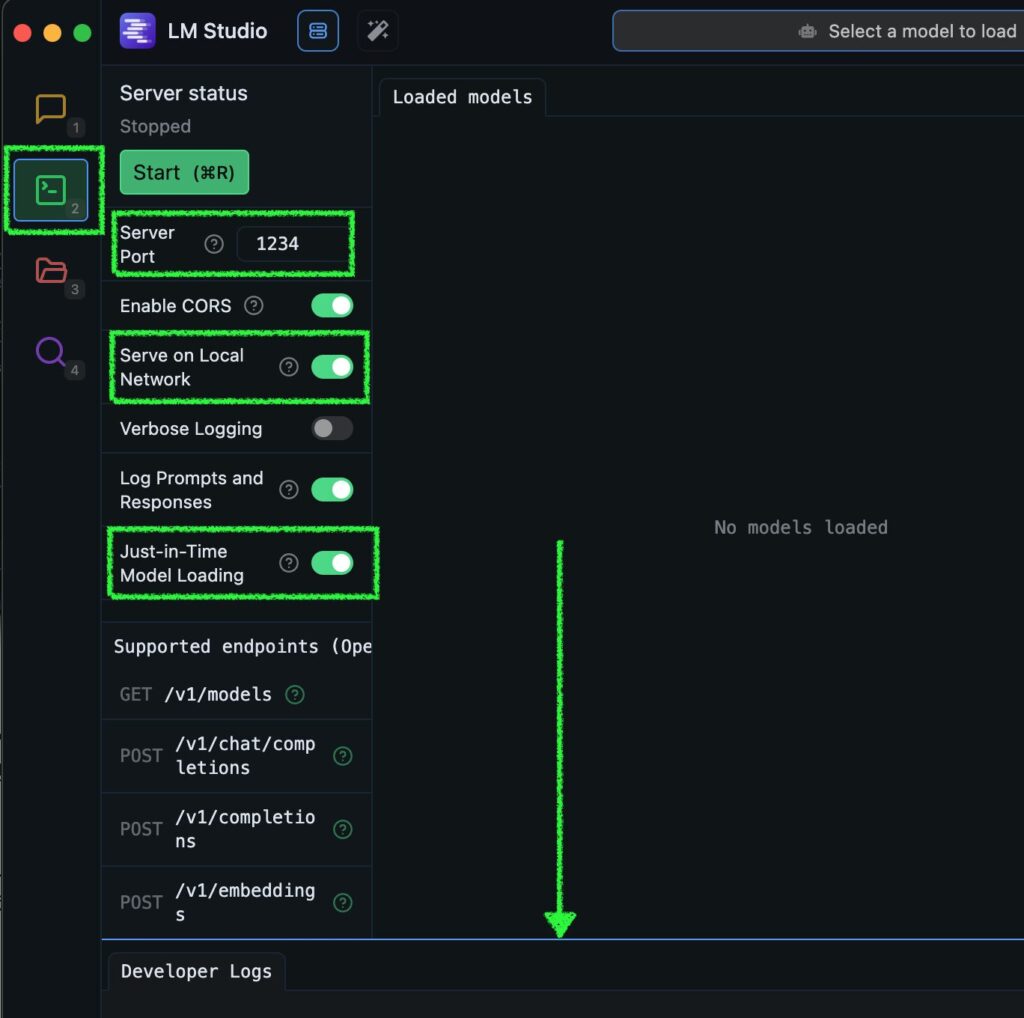
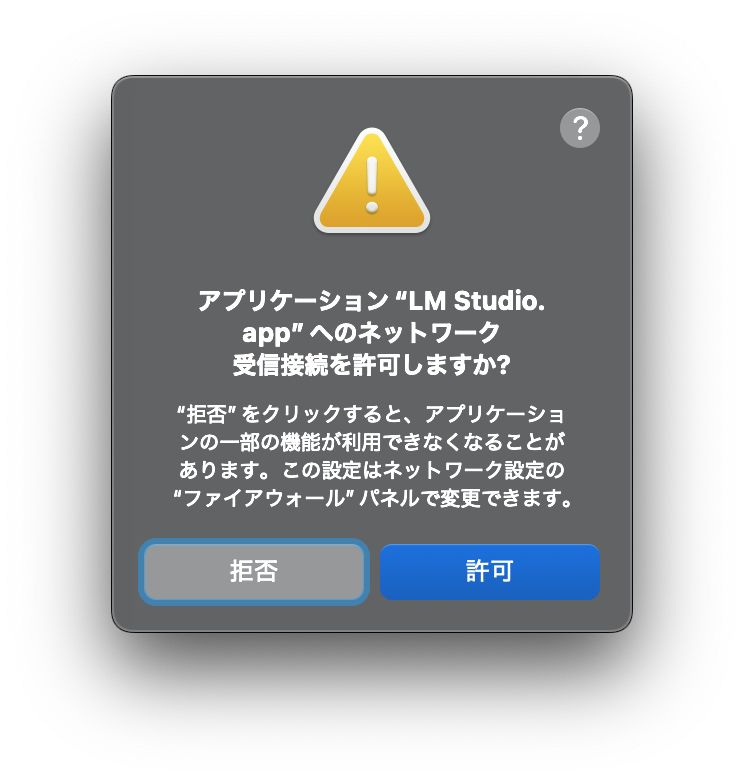
ヘッドレスモード (GUI 無し API サーバのみ)
右下のギアアイコンまたはコマンド+カンマで App Settings を開き、Local LLM Service (headless) を有効にします。これで、GUI を Quit しても API サーバは動き続けます。

GUI を開いたり、サーバを停止したりするには、メニューバーのアイコンを開きます。メモリを解放するなら、Unload All Models または、Quit LM Studio を選びましょう。
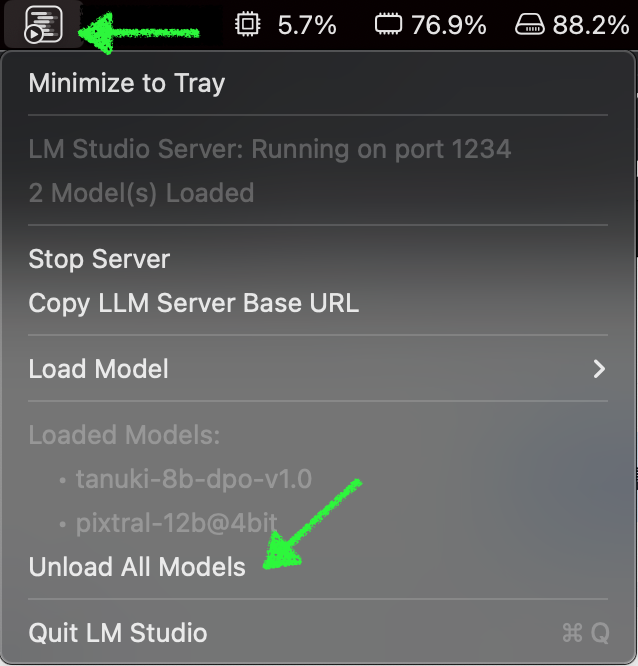
Dify から LM Studio API サーバに接続できるようにする
モデルプロバイダーにある、OpenAI-API-compatible に登録します。
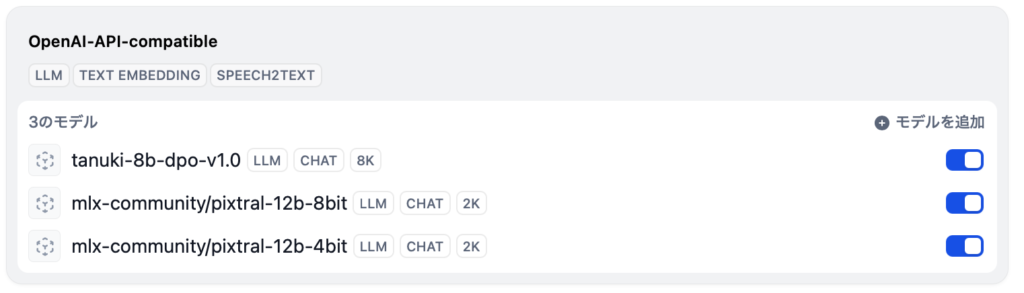
設定内容はこんな感じです:
- Model Name: モデル名 (例: mix-community/pixtral-12b-4bit)
- API Key: 無し
- API endpoint URL: 例 http://192.168.1.10:1234/v1 とか http://localhost:1234/v1 (ポート番号/v1 を忘れずに)
- Completion mode: Chat
- Model context size 2048
- Upper bound for max tokens: 2048
- Function calling: Not Support
- Streaming function calling: Support
- Vision Support: Support
- Delimiter for streaming results: \n\n (区切り文字が入力必須ですが、とりあえず改行二つのこれで動きます)
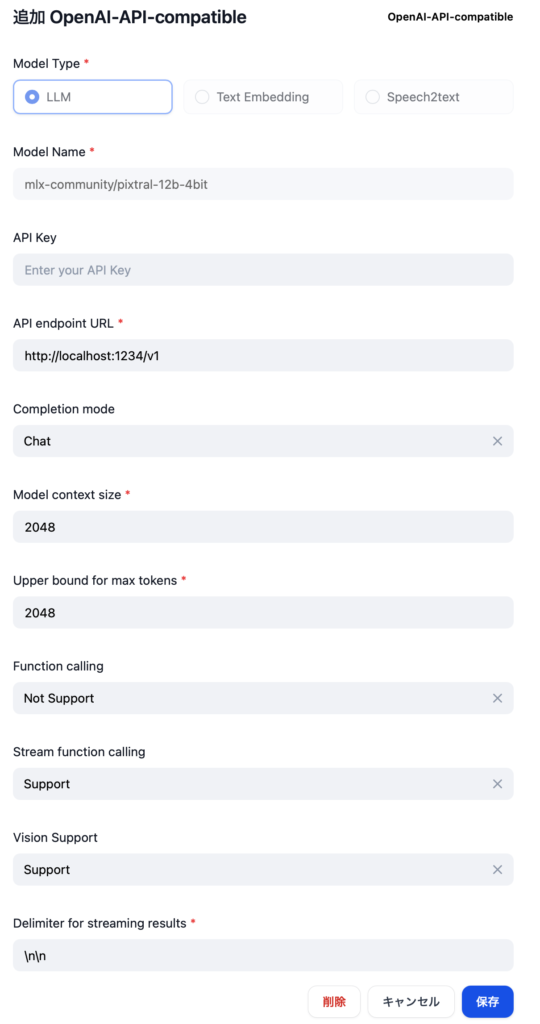
Dify で動く超シンプル画像認識アプリ
DSL ファイルを下に貼っておきますが、チャットボットの Chatflow を作り、機能で「画像アップロード」を有効にして、モデルとして Pixtral を選んでいるくらいの単純なものです。
DSL を開く
app:
description: 32GB RAM オンメモリで動作するビジョンモデル。Pixtral は MLX 版で、LM Studio で実行中。事前に LM Studio
サーバを実行しておくこと
icon: eyes
icon_background: ‘#D5F5F6’
mode: advanced-chat
name: Pixtral 12B 4Bit (MLX)
use_icon_as_answer_icon: true
kind: app
version: 0.1.2
workflow:
conversation_variables: []
environment_variables: []
features:
file_upload:
image:
enabled: true
number_limits: 3
transfer_methods:
– local_file
– remote_url
opening_statement: ”
retriever_resource:
enabled: false
sensitive_word_avoidance:
enabled: false
speech_to_text:
enabled: false
suggested_questions: []
suggested_questions_after_answer:
enabled: false
text_to_speech:
enabled: false
language: ”
voice: ”
graph:
edges:
– data:
isInIteration: false
sourceType: start
targetType: llm
id: 1730552831409-source-llm-target
source: ‘1730552831409’
sourceHandle: source
target: llm
targetHandle: target
type: custom
zIndex: 0
– data:
isInIteration: false
sourceType: llm
targetType: answer
id: llm-source-answer-target
source: llm
sourceHandle: source
target: answer
targetHandle: target
type: custom
zIndex: 0
nodes:
– data:
desc: ”
selected: false
title: 開始
type: start
variables: []
height: 54
id: ‘1730552831409’
position:
x: 30
y: 253.5
positionAbsolute:
x: 30
y: 253.5
selected: false
sourcePosition: right
targetPosition: left
type: custom
width: 244
– data:
context:
enabled: false
variable_selector: []
desc: ”
memory:
query_prompt_template: ‘{{#sys.query#}}’
role_prefix:
assistant: ”
user: ”
window:
enabled: false
size: 10
model:
completion_params:
temperature: 0.7
mode: chat
name: mlx-community/pixtral-12b-4bit
provider: openai_api_compatible
prompt_template:
– id: 9011766a-9f0d-45d2-87bb-8f2665ad80db
role: system
text: ”
selected: true
title: Vision
type: llm
variables: []
vision:
configs:
detail: high
enabled: true
height: 98
id: llm
position:
x: 334
y: 253.5
positionAbsolute:
x: 334
y: 253.5
selected: true
sourcePosition: right
targetPosition: left
type: custom
width: 244
– data:
answer: ‘{{#llm.text#}}’
desc: ”
selected: false
title: 回答
type: answer
variables: []
height: 107
id: answer
position:
x: 638
y: 253.5
positionAbsolute:
x: 638
y: 253.5
selected: false
sourcePosition: right
targetPosition: left
type: custom
width: 244
viewport:
x: 49.4306562198135
y: 352.30093613089844
zoom: 0.7237754523423506
アプリを公開するとチャットウィンドウに画像をドラッグアンドドロップできるので、画像と質問を入力すれば回答が返ってきます (初回の LLM のロードが行われる時はタイムアウトするかもしれません。少し待って再度コメントを投げると返事が来ます)。Dify から使うと若干スピードが落ち、何度か試した後確認すると、20 TPS 以下でした。実行時のメモリプレッシャーはこんな感じ ↓ です。モデルがロードされた後のメモリの使用量が、ロード前ほど下がらないことがわかると思います。この時で LM Studio Helper のメモリ使用量は 12GB ほどでした。

以前の記事で使った画像を読ませたところ ↓
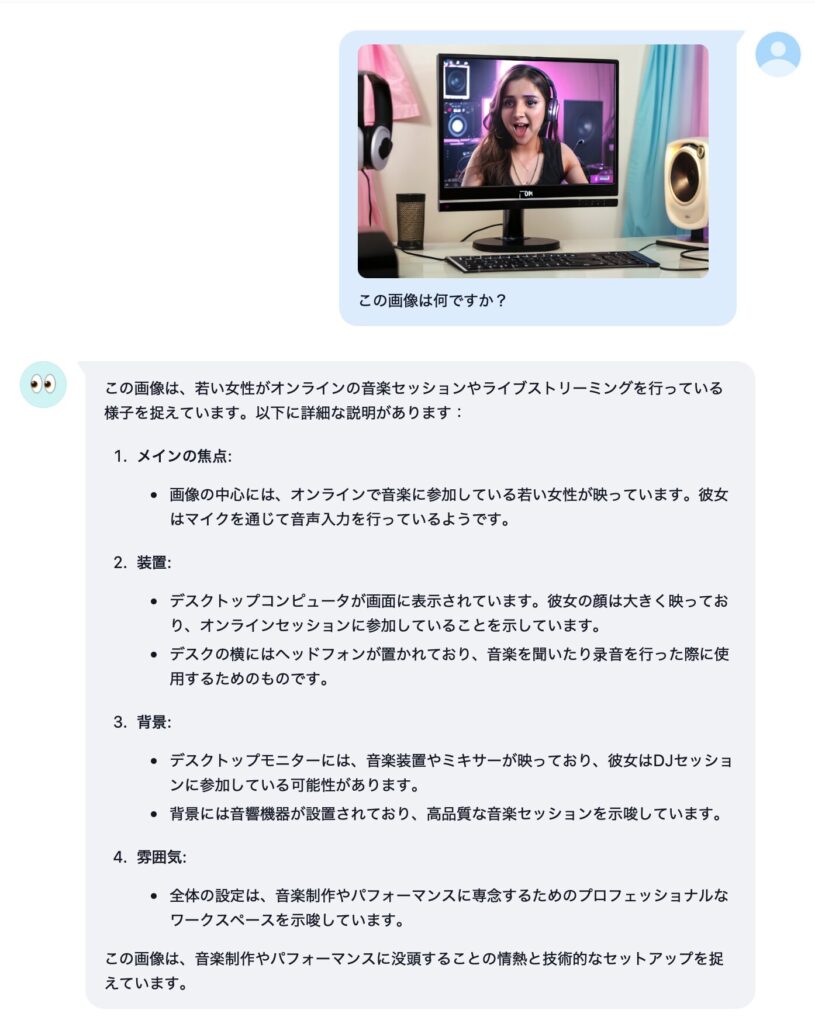
Pixtral さんは画像の中の日本語は読めない (日本語 OCR としては使えない)
チャットでは流ちょうな日本語でやりとりできるのですっかりその気になっていたのですが、画像の中の日本語は読めないみたいです。ベーマガのペーパー・アドベンチャーをスキャンして遊びやすくしようと思っていたのですが、そういう用途には使えないようです (注意: 雑誌のスキャンを無許可で公開すると著作権侵害となります)。
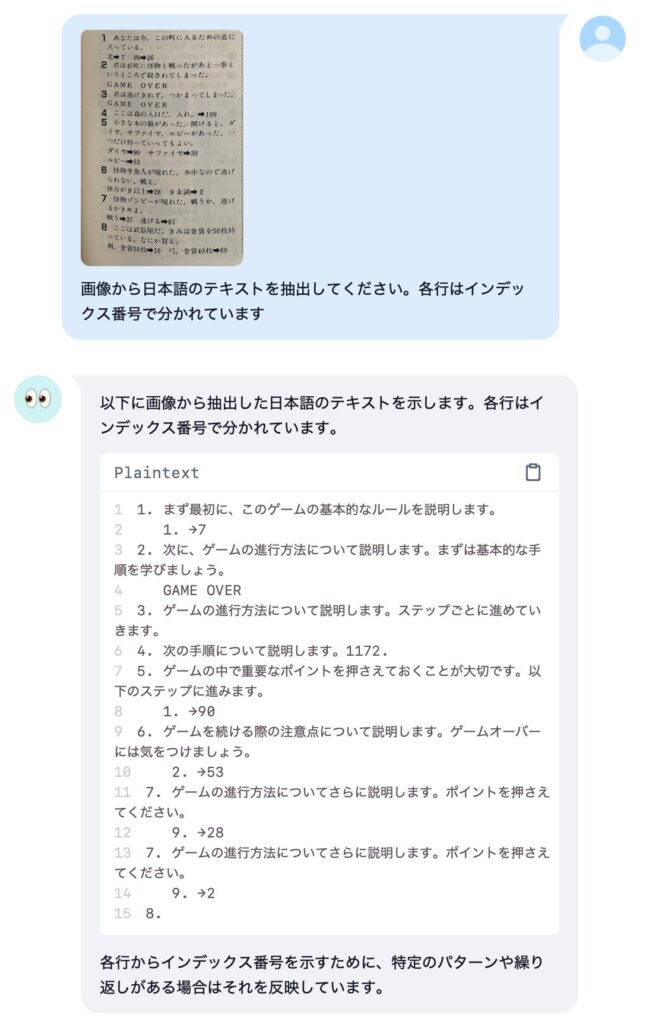
雷門が歌舞伎町に見えてしまえば、文字だってそう読めてしまいます。
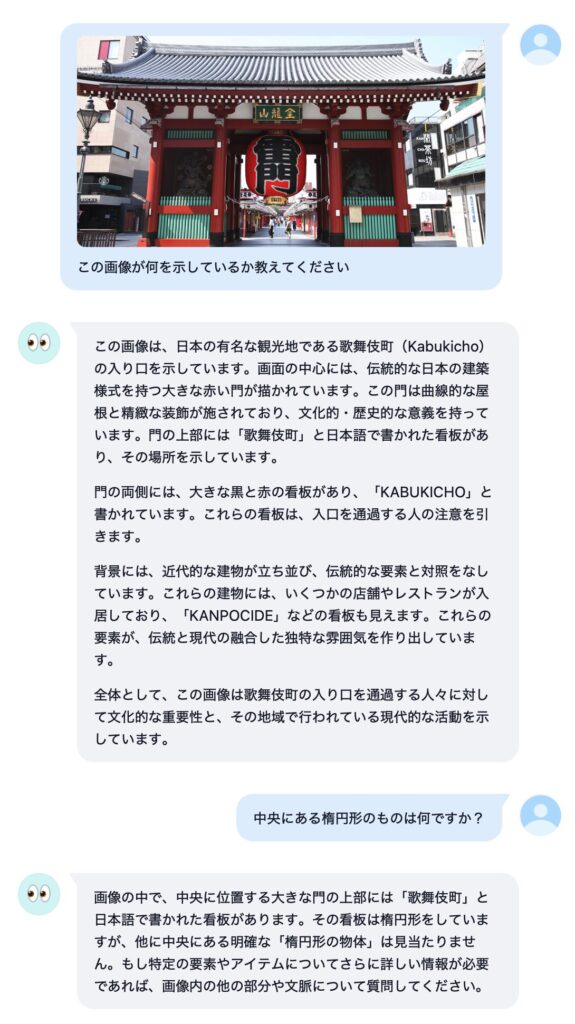
かわいい手書き文字でもテスト。うーむ、日本語 OCR として使うのは諦めた方がよさそうです。
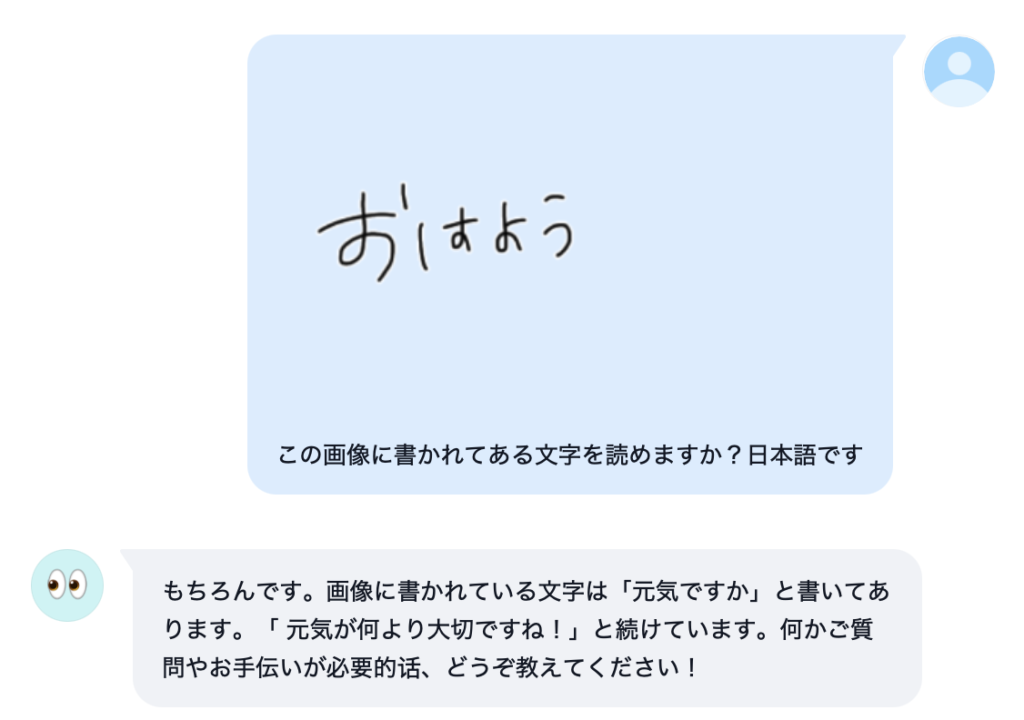
念のため英語とフラ語でも聞いてみました。
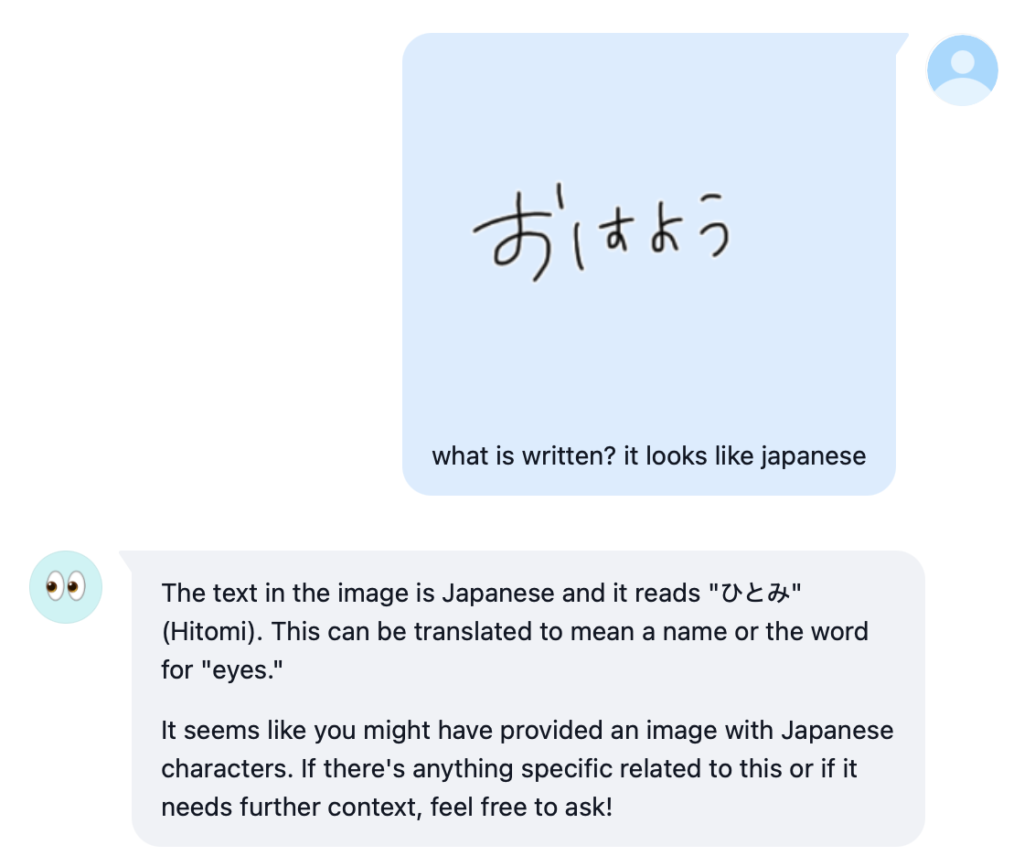
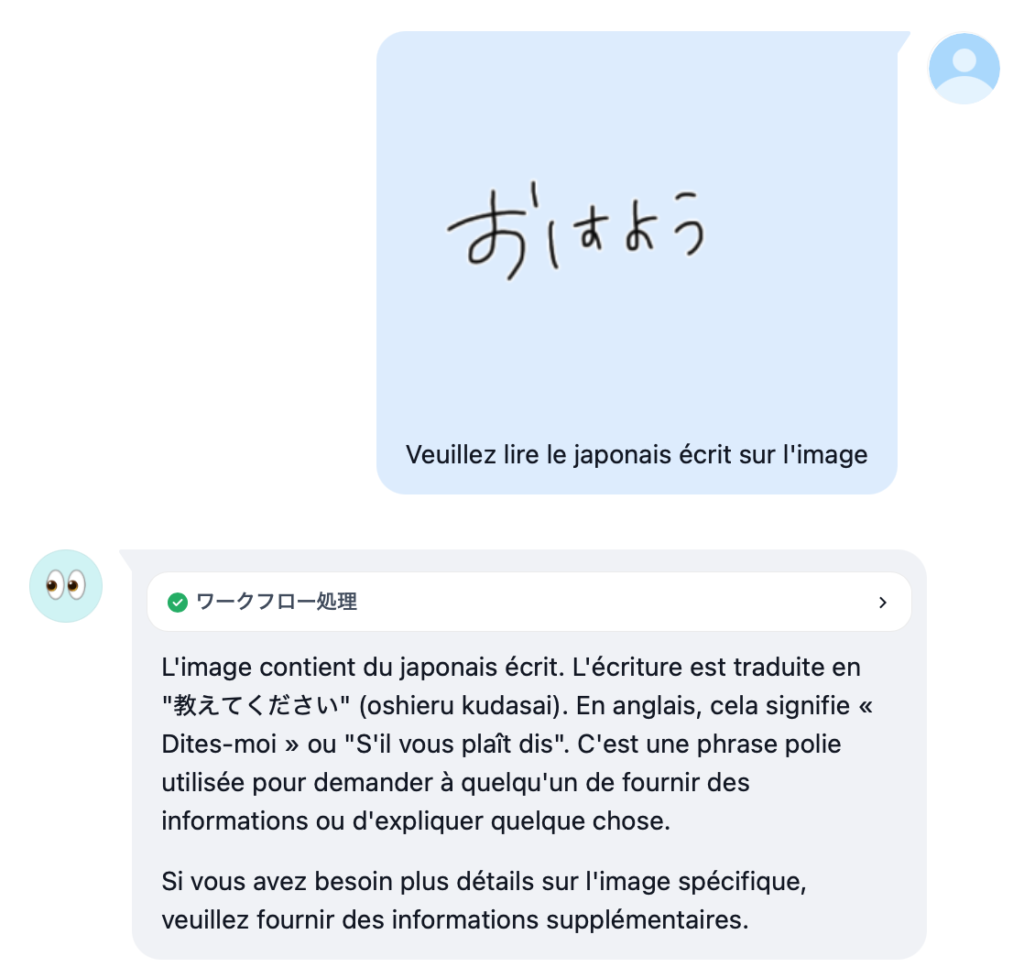
面白かったのでいくつも画像を貼ってしまいましたが、日本語 OCR 能力は無いという結論に変更はありません。アルファベットなら手書きもまーまーイケそうなので残念ですが、このパラメータ数 (12B) ですし日本語に特化しているわけでもないわけですから、欲しがりすぎてはいけませんね。
(おまけ) Mac で OCR するなら、写真の文字をなぞってコピーするだけ
話はそれますが、Mac なら画像や写真の文字部分をなぞればほぼコピーできちゃいます。素のままでできるはずですが、うまくいかないときはプレビューで開いて、ツールからテキスト選択選べばほぼイケるかと。
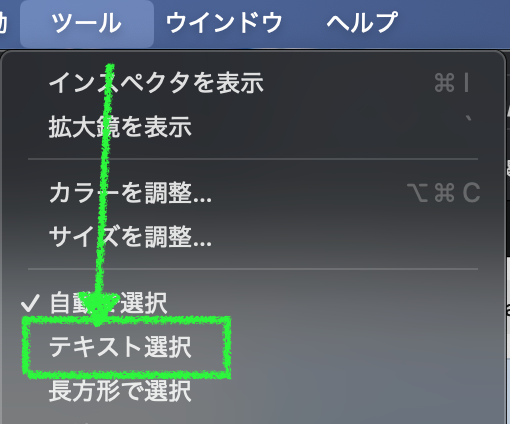
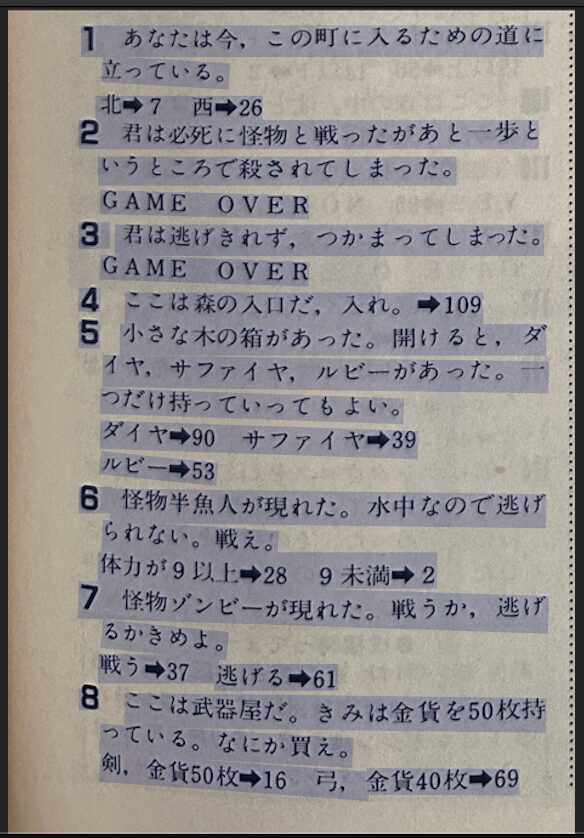
全文載せると著作権侵害になるので、冒頭部分のみ (関係者の方からご指摘あれば削除いたします)。適当に iPhone で撮影した写真でもこの認識力。改行位置の的確さもほぼ OK。これが OS レベルで実装されているのですごい。すごいありがたい macOS。
1 あなたは今、この町に入るための道に立っている。
北→7 西→26
2 君は必死に怪物と戦ったがあと一歩というところで殺されてしまった。
GAME OVER
3
君は逃げきれず、つかまってしまった。
GAME OVER
4
5
ここは森の入口だ、入れ。→109小さな木の箱があった。開けると、ダイヤ,サファイヤ、ルビーがあった。】
つだけ持っていってもよい。
ダイヤ→90 サファイヤ→39
ルビー→53
6
怪物半魚人が現れた。水中なので逃げられない。戦え。
体力が9以上→28 9未満→2
7怪物ゾンビーが現れた。戦うか,逃げ
るかさめよ。
戦う→37
逃げる→61
8
ここは武器屋だ。きみは金貨を50枚持っている。なにか買え。
剣,金貨50枚→16号,金貨40枚→69
Apple Inteligence もまもなく使えるようですし、AI スタートアップで収益上げるって難しそうだな、と思いました。
Image by Stable Diffusion (Mochi Diffusion)
おフランスをパリジェンヌが歩いているおしゃれな画像を期待して描いてもらいました。
Date:
2024年11月3日 15:13:32
Model:
realisticVision-v51VAE_original_768x512_cn
Size:
768 x 512
Include in Image:
masterpiece, intricate, realistic, sharp focus, photograph, woman walking in Paris
Exclude from Image:
Seed:
2526772693
Steps:
23
Guidance Scale:
20.0
Scheduler:
DPM-Solver++
ML Compute Unit:
CPU & GPU